Mastering HTML5 Offline Storage for Your Website
HTML5 introduces many new features, and one of the most exciting is Offline Storage. In this article, we’ll explore two key types of offline storage: sessionStorage and localStorage. Offline Storage allows us to save data directly in the user’s browser, enabling our web apps or games to function without an internet connection for a certain period of time.
In practical terms, developers can use Offline Storage as a backup mechanism when the Internet connection is unavailable. Once connectivity is restored, they can seamlessly sync the stored data with the online server.
If you’re wondering how to implement this powerful feature on your website, read on for a comprehensive guide.
MarsEdit: Offline WordPress Editor For Mac (Review)
A quick look at MarsEdit and its helpful features. Read more
SessionStorage
sessionStorage is a type of storage that temporarily holds data in the browser. This data is stored as key-value pairs and is specific to the browser window or tab. As long as the browser or tab remains open, the data persists unless we intentionally erase it or close the browser.
To store data in sessionStorage, we can use .setItem()
. Here’s an example where we store “Hello World”.
sessionStorage.setItem("keyExample", "Hello World");
Alternatively, we can also do the following, which creates a data entry with anotherKeyName
as the key and ‘Hello Too’ as the value.
sessionStorage.anotherKeyExample = "Hello Too";
In Webkit-based browsers like Safari, Chrome, and Opera, you can view the data under the Resources tab. In Firefox, it appears under the Firebug DOM tab.
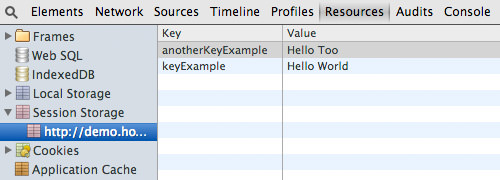
Note that sessionStorage can only store strings or plain text. An integer
will be converted to a string.
If you have JSON data, you need to format it as a string using JSON.stringify()
and retrieve it using JSON.parse()
to convert the string back to JSON. Here are a few examples:
var json = JSON.stringify([1, 2, 3]); sessionStorage.anotherKeyExample = json;
Retrieving Data in sessionStorage
We have two ways to retrieve data from sessionStorage. First, we can use .getItem()
or directly reference the key name, as shown below.
var a = sessionStorage.getItem("keyExample"); var b = sessionStorage.anotherKeyExample;
Deleting Data in sessionStorage
As mentioned, the data in sessionStorage is deleted when the user closes the browser window or tab. However, we can also delete it intentionally. We can use the .removeItem()
method or the delete
directive, like this:
sessionStorage.removeItem("keyExample"); delete sessionStorage.anotherKeyExample;
LocalStorage
localStorage allows us to store data in the browser persistently. Unlike sessionStorage, localStorage data remains in the browser until we intentionally remove it.
Storing data in localStorage is just as simple as in sessionStorage. The process is identical, except we use the localStorage
object. We can create a data entry using the .setItem()
method or by directly setting it with the key name, like this:
localStorage.setItem("keyName", "Hello, Local Storage"); localStorage.anotherKeyName = 1;
To retrieve data, we use the .getItem()
method.
var c = localStorage.getItem("keyName"); var d = localStorage.anotherKeyName;
Similarly, we can remove a data entry from localStorage using the .removeItem()
method or the delete
directive.
localStorage.removeItem("keyName"); delete localStorage.anotherKeyName;
Offline Storage Limit Size
Both sessionStorage and localStorage have limits in terms of maximum capacity, and each browser has its own limit. Firefox, Chrome, and Opera have a limit of 5MB per domain. Internet Explorer offers more space with 10MB per domain. Ensure that your data does not exceed these limits. If your data does exceed the limit, you might want to consider alternatives such as SQLite.
Feature Detection
Although support for sessionStorage and localStorage is quite extensive (even IE8 supports them), it is wise to perform browser feature detection before using these storage methods. This way, you can implement a fallback function, like using Cookies, in case the browser does not support Offline Storage.
You can use Modernizr for this purpose or wrap your script with a conditional statement like this:
if (window.localStorage) { // localStorage is available } else { alert('localStorage is not available'); }
Conclusion
Offline Storage is a fantastic feature that allows web apps and games to function offline. We’ve also demonstrated how to use it in real-world examples in the past.
Check these out: How To Use Cookie & HTML5 LocalStorage & Edit Web Content On Front-End.
I hope this short article helps you get started with Offline Storage.