Essential WordPress Plugins and Snippets to Improve Search Functionality
Editor’s note: For a newer, updated version of this post, check it out here.
WordPress is a powerful CMS that powers not only blogs but also countless forums and personal websites. While many features offered are advanced for the market, the search functionality still lags behind. The built-in search provides a simple solution for the complex problem of finding the right content on your site.
Although WordPress’s search function is adequate for finding articles based on direct matches, it falls short in several areas. For instance, it lacks the ability to search across all categories, tags, or within specific categories and tags. Additionally, search results are displayed by default in chronological order, from newest to oldest. This is a significant UX gap for users who may be looking for popular articles with the most views or comments.
In this post, I’ll provide an overview of WordPress search features and how they work within the system. Understanding the default setup will make customizing searches much easier. Additionally, I’ll introduce some powerful plugins and code snippets that are beneficial for any WordPress website.
Understanding the Basics of WordPress Search
When you perform a search query in WordPress, results are returned based on publication time, including pages – if WordPress is configured to do so. Two plugins, Search Unleashed and Search Everything, allow users to search through pages and comments as well. A significant drawback is that WordPress search does not leverage keywords effectively.
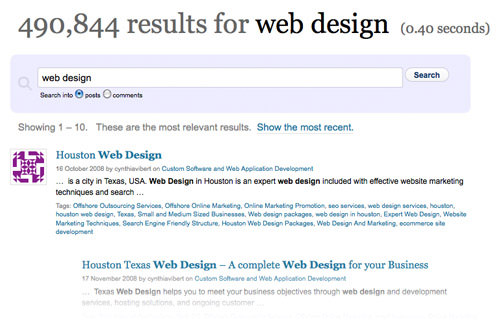
If an article was published a year or two ago, the chances of it being found in a search are slim unless the user enters specific keywords into a larger search engine like Google or Bing. When you search for “web design,” WordPress looks for an exact match for that phrase, ignoring results that simply contain “design.” This limitation significantly reduces the effectiveness of searches.
Categories and tags can also affect search results. WordPress’s search functionality is outdated compared to most other systems, but fortunately, it can be enhanced with the help of the development community.
Working with WordPress Theme Files
Each WordPress theme contains a set of files that manage search functionality and forms. The main template file for search results is search.php.
Some developers mistakenly include their search.php file inside another core file, such as page.php or single.php. While this can be effective for building modular templates, the standalone search file is used solely for displaying pagination and results. The standard file name searchform.php includes basic PHP code for handling search queries. The rest of the file is a simple HTML form with one input field and a submit button.
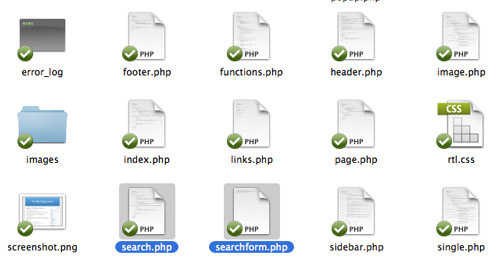
This file is often included in the header or sidebar area of templates, providing a ready-made form that users can utilize for search queries. HTML5 attributes allow you to include default text in the input field, such as “Search…” or “Enter terms here.”
To display your search form, you can use the get_search_form()
function, which can be added anywhere in your templates. This internal WordPress function simplifies the development of search functionality.
Using the WP Query Function
WordPress includes a powerful function called WP_query()
that allows developers to create custom SQL queries. This function is often used by developers and theme designers to create more complex search queries than what WordPress provides by default.
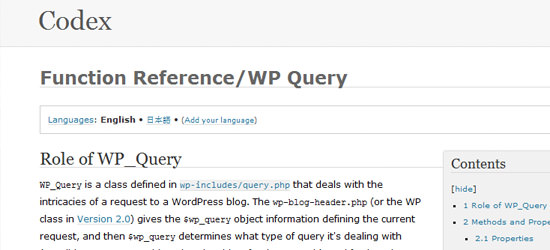
If you’re a developer, I recommend reviewing the WP_Query function reference for detailed insights. Although the documentation is extensive, it offers valuable features, such as pulling specific posts or categories based on the current page content.
The Query function also allows you to check against the current page value. WordPress automatically assigns a name to each page type on your site, such as blog posts, pages, search results, and homepages. Below is a list of common page variables for those interested in diving deeper.
$is_single
– Viewing a single post page$is_author
– Viewing an author post directory page$is_search
– Viewing a search results page$is_category
–$is_tag
– Viewing a list of posts by category or tag$is_404
– Viewing a 404 error page
WordPress Search Plugins
Here are some popular plugins related to search and queries. All of them are free and available for download from the official WordPress plugin directory. However, avoid installing more than 2 or 3 at a time – read the descriptions and test them individually to find the best fit for your blog.
Search Meter
Search Meter is a valuable tool for webmasters interested in tracking search analytics. It stores every search query in the admin panel, providing detailed insights into search performance. You’ll receive data on failed searches, popular search terms, and more. The plugin generates statistics that you can reset or export for further analysis.
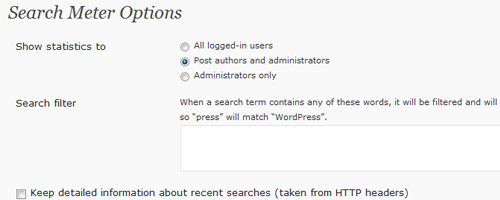
Highlight Search Terms
This plugin is widely used across many blogs today. When a visitor finds your page through a search engine (e.g., Google, Yahoo!, Bing), the search keywords are highlighted within your content. This helps visitors quickly locate relevant information. Note that the plugin does not include default CSS styles, so you’ll need to design them yourself after activation.
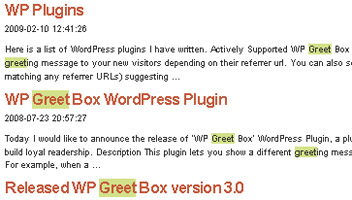
Better Search
As the name suggests, Better Search is a plugin designed to improve WordPress’s search functionality. It enhances search results by considering keyword relevancy, meta tags, post tags, and categories. The search form also displays the most popular search terms on your blog, which is updated frequently based on search traffic.
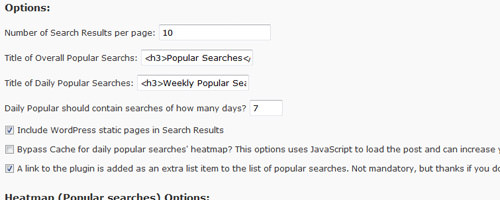
WordPress Search Snippets
1. Exclude Posts/Pages from Search Results
This function allows you to exclude posts from specific categories or even pages from search results. (via wprecipes)
Insert in: functions.php
function SearchFilter($query) { if ($query->is_search) { $query->set('cat', '0,1'); } return $query; } add_filter('pre_get_posts', 'SearchFilter');
2. Search Within a Specific Category
This snippet allows you to return search results from a specific category.
Insert in: functions.php
function SearchFilter($query) { if ($query->is_search) { // Insert the specific categories you want to search $query->set('cat', '8,9,12'); } return $query; } add_filter('pre_get_posts', 'SearchFilter');
3. Search Within a Specific Post Type
Use this function to filter out all other post types and target your search to a specific WordPress post type.
Insert in: functions.php
function SearchFilter($query) { if ($query->is_search) { // Insert the specific post type you want to search $query->set('post_type', 'feeds'); } return $query; } // This filter will jump into the loop and arrange our results before they're returned add_filter('pre_get_posts', 'SearchFilter');
4. Highlight WordPress Search Terms (jQuery)
This snippet highlights search terms on the WordPress search results page. (via weblogtoolscollection)
Insert in: functions.php
function hls_set_query() { $query = attribute_escape(get_search_query()); if(strlen($query) > 0){ echo ' '; } } function hls_init_jquery() { wp_enqueue_script('jquery'); } add_action('init', 'hls_init_jquery'); add_action('wp_print_scripts', 'hls_set_query');
Insert in: header.php (before </head>)
<style type="text/css" media="screen"> .hls { background: #D3E18A; } </style> <script type="text/javascript"> jQuery.fn.extend({ highlight: function(search, insensitive, hls_class){ var regex = new RegExp("(<[^>]*>)|(\\b" + search.replace(/([-.*+?^${}()|[\]\/\\])/g,"\\$1") + ")", insensitive ? "ig" : "g"); return this.html(this.html().replace(regex, function(a, b, c){ return (a.charAt(0) == "<") ? a : "<strong class=\"" + hls_class + "\">" + c + ""; })); } }); jQuery(document).ready(function($){ if(typeof(hls_query) != 'undefined'){ $("#post-area").highlight(hls_query, 1, "hls"); } }); </script>
5. Display Search Term + Result Count
This snippet returns search queries and the number of results. Example: Search Result for “twitter” – 8 articles. (via wpbeginner)
<h2 class="pagetitle">Search Result for</h2>
<?php
$allsearch = &new WP_Query("s=$s&showposts=-1");
$key = wp_specialchars($s, 1);
$count = $allsearch->post_count;
_e(''); _e('');
echo $key; _e(''); _e(' — ');
echo $count . ' '; _e('articles'); wp_reset_query();
?>