Creating a Stitched Effect Using CSS
There are many visual effect possibilities we can achieve with CSS; the limit is only our creativity and imagination. This time, as the title suggests, we are going to try creating the stitched effect using only CSS.
Let’s get started.
Understanding Pseudo-Element :before and :after
Cascading Style Sheet (CSS) is primarily intended for applying styles to the HTML markup, however in some cases... Read more
Preparing the Assets
First, we need resources to deliver the F icon of Facebook. In this tutorial, we are going to use this font: Modern Pictogram by John Caserta. We will also need an image for the background, and this time, we are going to use this linen texture from Premium Pixels.
Then, put all these things in appropriate folders, like so;
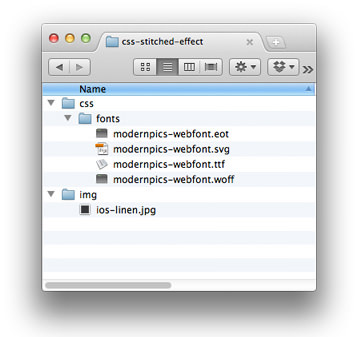
Structuring HTML Markup
Create a new HTML document and CSS file. Then, link the CSS file as well as the CSS Normalize in the <head>
section, as follows.
<link rel="stylesheet" href="https://github.com/necolas/normalize.css/2.0.1/normalize.css"> <link rel="stylesheet" href="css/style.css">
To create this icon, we only need a single div
. Put this div
below within the <body>
section.
<div class="icon">F</div>
Styles
Now, we work on the styles and, as usual, we start off by adding the @font-face
rule and add the linen background in the body
document, like so.
@font-face { font-family: 'ModernPictogramsNormal'; src: url('fonts/modernpics-webfont.eot'); src: url('fonts/modernpics-webfont.eot?#iefix') format('embedded-opentype'), url('fonts/modernpics-webfont.woff') format('woff'), url('fonts/modernpics-webfont.ttf') format('truetype'), url('fonts/modernpics-webfont.svg#ModernPictogramsNormal') format('svg'); font-weight: normal; font-style: normal; } body { background: url('../img/ios-linen.jpg'); }
Then, we add decorative styles to .icon
, including specifying the height
and width
, add rounded corners, box shadow, and color gradient for the background.
.icon { font-family: "ModernPictogramsNormal"; font-size: 4em; color: #fff; text-align: center; line-height: 0.406em; text-shadow: 1px 1px 0px rgba(0, 0, 0, .5); width: 65px; height: 65px; padding: 7px; margin: 50px auto; position: relative; -webkit-border-radius: 6px; -moz-border-radius: 6px; border-radius: 6px; -webkit-box-shadow: 0px 0px 10px 1px rgba(0, 0, 0, .3), inset 0px 1px 0px 0px rgba(255, 255, 255, .15); -moz-box-shadow: 0px 0px 10px 1px rgba(0, 0, 0, .3), inset 0px 1px 0px 0px rgba(255, 255, 255, .15); box-shadow: 0px 0px 10px 1px rgba(0, 0, 0, .3), inset 0px 1px 0px 0px rgba(255, 255, 255, .15); background: #375a9a; background: -moz-linear-gradient(top, #375a9a 0%, #1b458e 100%); background: -webkit-gradient(linear, left top, left bottom, color-stop(0%, #375a9a), color-stop(100%, #1b458e)); background: -webkit-linear-gradient(top, #375a9a 0%, #1b458e 100%); background: -o-linear-gradient(top, #375a9a 0%, #1b458e 100%); background: -ms-linear-gradient(top, #375a9a 0%, #1b458e 100%); background: linear-gradient(to bottom, #375a9a 0%, #1b458e 100%); filter: progid: DXImageTransform.Microsoft.gradient(startColorstr='#375a9a', endColorstr='#1b458e', GradientType=0); } .icon:before, .icon:after { content: ""; display: block; width: 63px; height: 63px; -webkit-border-radius: 3px; -moz-border-radius: 3px; border-radius: 3px; position: absolute; }
Then, we add these pseudo-elements with dashed border, but each will have a different color. The :before
will be in dark blue, like so.
.icon:before { border: 1px dashed #0d2b5e; }
Whilst the :after
pseudo-element border will have white color with low intensity, we define it with RGBA color mode. Furthermore, we also need to position the :after
element 1px
from the top and 1px
from the left, so its border line is noticable.
.icon:after { border: 1px dashed rgba(255, 255, 255, 0.1); top: 7px; left: 7px; margin-top: 1px; margin-left: 1px; }
That’s all the codes we need. Here is the result and you can download the source from these links below.