Enhance Checkbox and Radio Button Design Using CSS3
With CSS3, we can customize web presentations to be almost anything we want them to be. In this post, we’ll explore how to personalize the appearance of checkbox
and radio
inputs using CSS.
These form elements can be tricky to style consistently across different platforms – Windows, macOS, and Linux each have their own default designs. This tutorial will guide you through customizing these elements for a unified appearance.
If you’re looking to personalize these form elements, this tutorial is for you. Let’s dive in.
HTML Markup
We’ll start by creating a basic HTML structure.
Radio Input
<div class="radio"> <input id="male" type="radio" name="gender" value="male"> <label for="male">Male</label> <input id="female" type="radio" name="gender" value="female"> <label for="female">Female</label> </div>
Checkbox Input
<div class="checkbox"> <input id="check1" type="checkbox" name="check" value="check1"> <label for="check1">Checkbox No. 1</label> <br> <input id="check2" type="checkbox" name="check" value="check2"> <label for="check2">Checkbox No. 2</label> </div>
CSS
With the HTML structure in place, let’s add some styles to the <input>
elements. We’ll start with the radio input type. The goal is to transform the default OS design into something similar to the screenshot below.
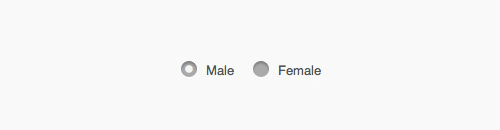
Styling Radio Input
First, we’ll set the cursor on the labels to a pointer to indicate they are clickable, and then apply some additional styles.
label { display: inline-block; cursor: pointer; position: relative; padding-left: 25px; margin-right: 15px; font-size: 13px; }
Next, we’ll hide the default radio
input.
input[type=radio] { display: none; }
We’ll then replace the hidden radio
input with a pseudo-element using :before
.
label:before { content: ""; display: inline-block; width: 16px; height: 16px; margin-right: 10px; position: absolute; left: 0; bottom: 1px; background-color: #aaa; box-shadow: inset 0px 2px 3px rgba(0, 0, 0, .3), 0px 1px rgba(255, 255, 255, .8); }
The above styles can also be applied to the checkbox input type. The only difference is that the radio input type is circular. To achieve this, we add border-radius
with a value that’s half the height and width.
.radio label:before { border-radius: 8px; }
Recommended Reading: Understanding Pseudo-elements: before and after
At this stage, here’s how our radio
input type looks:
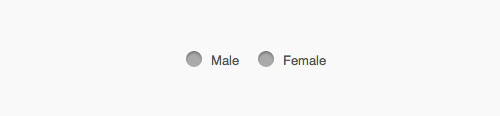
Now, we’ll add a small circle inside when the input is checked. We will utilize the CSS3 :checked
pseudo-class along with the Bullet HTML character (•
). To use this character in CSS, you’ll need to convert it using an Entity Conversion Tool.
input[type=radio]:checked + label:before { content: "\2022"; color: #f3f3f3; font-size: 30px; text-align: center; line-height: 18px; }
When the hidden radio input is checked, a smaller circle appears, as shown below.
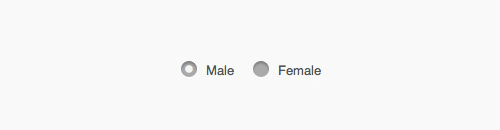
Alternatively, you can use an image as a background in the :before
pseudo-element instead of relying on CSS, but it’s often better to avoid images when possible.
Styling Checkbox Input
Next, we’ll style the checkbox
input type. As before, we’ll start by hiding the input element.
input[type=checkbox] { display: none; }
Since we’ve already replaced the input with a :before
pseudo-element, we’ll now add the border radius to create a square shape.
.checkbox label:before { border-radius: 3px; }
We’ll then add a checkmark using the Check Mark HTML Character (✓
) when the input is checked.
input[type=checkbox]:checked + label:before { content: "\2713"; text-shadow: 1px 1px 1px rgba(0, 0, 0, .2); font-size: 15px; color: #f3f3f3; text-align: center; line-height: 15px; }
Here’s the final appearance of our checkbox:
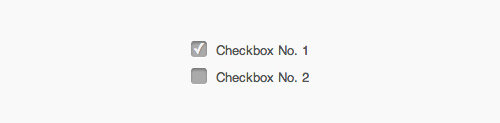
Final Thoughts
This is just one method to customize checkbox
and radio
inputs, and you can certainly enhance the look further. Keep in mind that this technique relies on CSS3 :checked
, so it will only work in browsers that support this feature. If you prefer, you can use a jQuery Plugin instead.
Finally, you can view the demo and download the source code using the links below: