How to Render Templates in Flask
Flask is a lightweight web framework for Python that makes it easy to build web applications. In our previous article, we’ve seen how to set up a simple page.
But, it’s just a simple text like “Hello Flask!”. In real applications, you’ll want to render more than just simple text. You’ll want to render HTML templates.
In Flask, we can do so with Jinja.
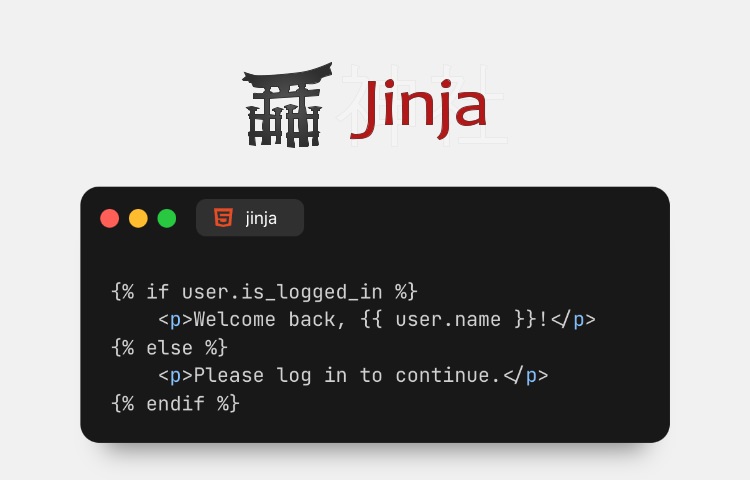
Jinja
One of its powerful features is the ability to render HTML templates using the Jinja templating engine.
Jinja templates are written in regular HTML that you are already familiar with. But, on top of that, you can use special syntax to handle dynamic content, such as for passing data from requests or other code sources to the template. It also allows you to use control structures like loops and conditionals.
Let’s see how it works in its basic form:
<!DOCTYPE html> <html> <head> <title>Welcome to Flask</title> </head> <body> {% if user.is_logged_in %} <p>Welcome back, {{ user.name }}!</p> {% else %} <p>Please log in to continue.</p> {% endif %} </body> </html>
It looks like a regular HTML file, but as we can see, we use some special syntax, such as the {{ }}
to insert a variable, and we use {% %}
for adding loops and conditionals.
Rendering the Templates
To render the template, we need to put it in the templates
directory.
. |-- app.py |-- templates |-- about.html |-- index.html
Then, we use the render_template
function in our Python app file to render HTML templates. If we extend the same code from our previous article, our code would now look like the following:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def home(): return render_template('index.html') @app.route('/about') def about(): return render_template('about.html')
Passing Variables
In our template above, we have {{ name }}
and {{ user.is_logged_in }}
variables. But, we haven’t passed any variables to the template yet.
To pass variables to the template, we can do so by passing them as arguments to the render_template
function.
For example:
@app.route('/') def home(): return render_template('index.html', user={'is_logged_in': True, 'name': 'Joann'})
Now, when we visit the home page, we will see the “Welcome back, Joann!” message instead of “Please log in to continue.” because the is_logged_in
is set to True
.
Template Inheritance
Jinja allows you to use template inheritance, which is helpful when multiple pages share the same layout or components. Instead of repeating common elements, we can create a base template and reuse it in other templates.
In this example, we have a homepage and an about page that share the same layout, like the head
, title
, and maybe some styles or scripts later on. With template inheritance, we can make things simpler and more organized.
First, we create the base file. Let’s name it base.html
. Then, add the common elements:
<!DOCTYPE html> <html> <head> <title>{% block title %}Welcome to Flask{% endblock %}</title> </head> <body> {% block content %}{% endblock %} </body> </html>
In this template, there are two blocks: one for the title and one for the content. The {% block %}
tag allows child templates to override specific sections of the base template.
Now, we can rewrite the index.html
file to extend the base.html
file. We can remove all of the basic HTML structure, like the head
and title
tags from the index.html
file, and replace it with the extends
tag.
{% extends 'base.html' %} {% block title %}Welcome to Flask{% endblock %} {% block content %} {% if user.is_logged_in %} <p>Welcome back, {{ user.name }}!</p> {% else %} <p>Please log in to continue.</p> {% endif %} {% endblock %}
On the about page, we could also rewrite it as follows:
{% extends 'base.html' %} {% block title %}About Us{% endblock %} {% block content %} <p>This is the about page.</p> {% endblock %}
When we visit the homepage, we’ll see the “Welcome back, Joann!” message if the user is logged in. On the about page, we’ll see “This is the about page.”. The difference here is that both pages are cleaner and easier to maintain, without repeating code.
Wrapping Up
That’s how we can render HTML templates in Flask using Jinja. Jinja is a powerful templating engine that comes with helpful features and extensions, making it easier to build dynamic web applications in Python.
There’s still a lot more to explore, but I hope this article gives you a solid starting point to get up and running with Flask.