Introduction to FrameworkX
PHP has come a long way and continues to improve with new features, syntax, and speed. The ecosystem is also expanding, with many developers creating frameworks to simplify the lives of other developers. Popular, full-featured frameworks like Laravel and Symfony exist, as do lightweight microframeworks like FrameworkX.
It is a lightweight microframework for PHP that uses an event-driven, non-blocking architecture, similar to Node.js which is perfect for high-concurrency and real-time applications like chat apps or live notifications.
In this article, we will explore what FrameworkX is and how it differs from traditional PHP frameworks. Let’s get started!
Getting Started
First, make sure you have PHP and Composer set up on your computer. Once installed, you can add FrameworkX to your project with this command:
composer require clue/framework-x
FrameworkX doesn’t require a complex setup. All you need is a public/index.php file. Here’s a basic example to display “Hello World!” on the homepage:
<?php require dirname(__DIR__) . '/vendor/autoload.php'; $app = new FrameworkX\App(); $app->get('/', fn () => \React\Http\Message\Response::plaintext("Hello world!\n")); $app->run();
To run your application, type:
php public/index.php
This command starts a local server using PHP’s built-in server, backed by the ReactPHP Socket component. No need for Nginx or Apache. Your server will run at http://127.0.0.1:8080
, and it should display “Hello World!”.
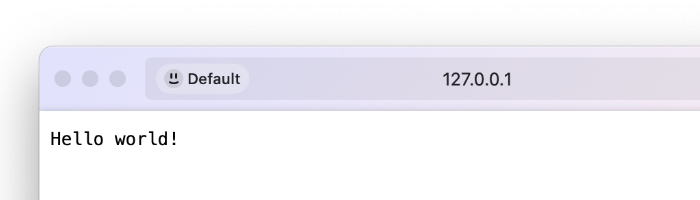
Besides plain text, you can also return JSON data. For example:
<?php require dirname(__DIR__) . '/vendor/autoload.php'; $users = [['name' => 'Jon Doe'], ['name' => 'Jane Doe']]; $app = new FrameworkX\App(); $app->get('/', fn () => \React\Http\Message\Response::json($users)); $app->run();
Async Operations
PHP operations are usually blocking and synchronous, meaning each task must finish before the next one starts. FrameworkX is built on the ReactPHP library.
ReactPHP is a library that provides components such as the EventLoop, Stream, Promise, Async, and HTTP components, which enable asynchronous operations. Thus, tasks can run concurrently without waiting for others to finish. This is ideal for handling multiple connections, HTTP requests, or I/O operations simultaneously.
In this example, we’ve updated our index.php to fetch an API. Instead of using the curl_*
functions, we will use the HTTP component to make an asynchronous request.
$app = new FrameworkX\App(); $app->get('/', function () { echo "Start\n"; (new \React\Http\Browser()) ->get('https://www.hongkiat.com/blog/wp-json/wp/v2/posts') ->then(function () { echo "End (API)\n"; }); echo "End\n"; return \React\Http\Message\Response::plaintext("Hello world!\n"); }); $app->run();
Normally, an external API request would block the page from rendering until the request completes. However, with the asynchronous operations that the ReactPHP HTTP component handles, the page loads instantly, as evidenced by the log.
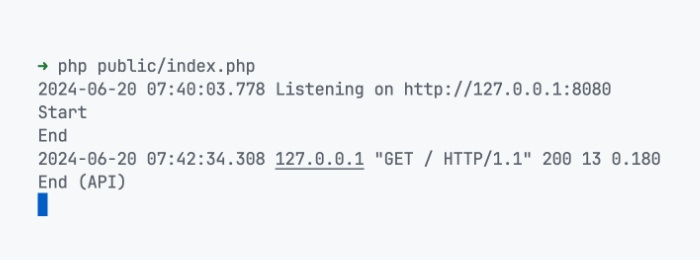
This makes FrameworkX capable of handling many more concurrent requests than traditional PHP setups, significantly speeding up page load times. But how fast is it?
Speed
I tested FrameworkX on a basic, inexpensive DigitalOcean droplet with 1 vCPU and 1GB of RAM. It handled around 4,000 requests per second effortlessly.
Concurrency Level: 50 Time taken for tests: 22.636 seconds Complete requests: 100000 Failed requests: 0 Keep-Alive requests: 100000 Total transferred: 17400000 bytes HTML transferred: 1300000 bytes Requests per second: 4417.69 [#/sec] (mean) Time per request: 11.318 [ms] (mean) Time per request: 0.226 [ms] (mean, across all concurrent requests) Transfer rate: 750.66 [Kbytes/sec] received
Even with additional workload, like disk read operations and rendering 100 lists from a JSON file, it still managed around 2700 requests per second.
Concurrency Level: 50 Time taken for tests: 36.381 seconds Complete requests: 100000 Failed requests: 0 Keep-Alive requests: 100000 Total transferred: 296700000 bytes HTML transferred: 280500000 bytes Requests per second: 2748.72 [#/sec] (mean) Time per request: 18.190 [ms] (mean) Time per request: 0.364 [ms] (mean, across all concurrent requests) Transfer rate: 7964.31 [Kbytes/sec] received
I’m pretty sure it could be much faster with better server specifications.
Wrapping Up
FrameworkX is a powerful, lightweight microframework for PHP. It runs asynchronously and is capable of handling multiple tasks efficiently, similar to Node.js. It’s the perfect framework whether you’re building a simple app or complex high-concurrency or real-time applications.