Getting Started with Flask
Flask is a lightweight and flexible micro-framework for Python that makes it easy to get started with web development. It’s designed to be simple and minimalistic, offering essential tools and features needed for building a web application, while allowing developers to have full control over how to implement additional features.
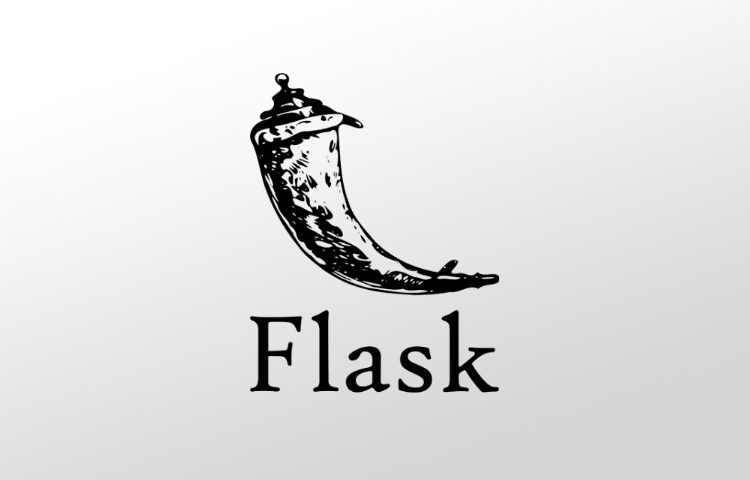
It is a “micro-framework,” which means it doesn’t require specific tools or libraries. This gives developers the freedom to decide how they want to extend their application, making Flask a good choice for those who prefer flexibility and customization. If you’re coming from PHP, this could be an alternative to using other micro-frameworks like Slim. If you’re coming from Ruby, you might find Flask similar to Sinatra.
Let’s see how we can get started with Flask and build a simple web page.
Install Flask
First, we need to install Flask. We can do this by running:
pip install flask
Create the App File
Next, we need to create a Python file, for example, app.py
. To get started, we add the following code:
from flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello Flask!"
The Flask
class is used to create an instance of the app. The @app.route('/')
decorator maps the homepage URL, /
, to the home
function, which returns the message “Hello Flask!”.
Run the App
Now, run the app using the following command:
flask run --debug
When we visit http://127.0.0.1:5000/
in the web browser, we’ll see the message “Hello Flask!”.
In this case, we also run the app in debug mode, which automatically reloads the server when you make changes to the code. This is useful for development, as you can see the changes immediately without having to restart the server. Keep in mind that you’d want to disable debug mode when deploying your application to a production server.
Routing
One of the main features provided by Flask is routing. Routing is the process of mapping URLs to functions that handle requests. In the example above, we defined a route for the homepage using the @app.route('/')
decorator. This tells Flask to call the home
function when the user visits the homepage URL, /
.
We can define routes for other pages as well. For example, to create an About
page, we can add the following code:
@app.route('/about') def about(): return "This is the About page."
Now, if we load http://127.0.0.1:5000/about
, we’ll see the message “This is the About page.”.
Wrapping up
Flask is a great choice for both beginners and experienced developers, especially when you want flexibility without the complexity of larger frameworks like Django.
In this article, we’ve covered the basics of how Flask works with some simple examples such as how to spin up the development server, and we learned a bit about routing in Flask to create static pages. In future articles, we’ll explore more advanced topics like rendering templates, working with forms, handling requests, and connecting to databases.
So stay tuned!