How to Handle HTTP Requests in Flask
In our previous article, we covered how to create simple pages in Flask and use Jinja2 as the templating engine. Now, let’s explore how Flask handles requests.
Understanding how HTTP requests work and how to manage them in Flask is key, as this allows you to build more interactive and dynamic web apps, such as building a form, API endpoints, and handling file uploads.
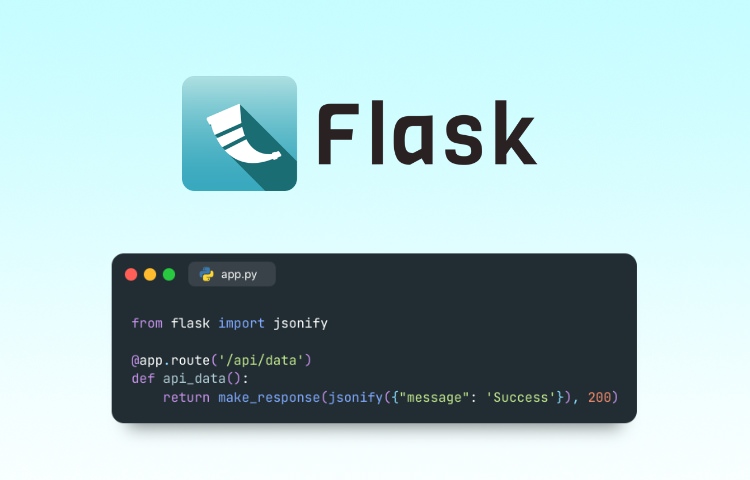
Without further ado, let’s get started.
So, What’s an HTTP Request?
An HTTP request is a message sent, usually by a browser, to the server asking for data or to perform an action. For example, when you visit a webpage, your browser sends a GET request to the server to retrieve the page’s content.
There are several different types of HTTP requests, and Flask can handle all of them, including GET
to retrieve data, POST
to send data to the server like submitting a form, PUT
to update existing data on the server, and DELETE
to delete data from the server.
Handling Requests in Flask
Flask makes handling requests straightforward by using routes. In our previous articles, we used routes to create static and dynamic pages. By default, routes only respond to GET
requests, but you can easily handle other HTTP methods by specifying them in the route.
Assuming we have a contact page at /contact
, we probably would want the page to handle both GET
and POST
requests to allow users to load the page, as well as to submit the form. To make the page handle these two HTTP methods, we can pass in the methods
argument, for example:
@app.route('/contact', methods=['GET', 'POST']) def submit(): if request.method == 'POST': data = request.form['input_data'] return render_template('contact.html', data=data) return render_template('contact.html')
In this example, users can load the /contact
page. When the form is submitted, Flask retrieves the form data and passes it to the contact.html
template. Then, within the template, you can access and process the data using Jinja2 templating.
Working with Query Parameters
Data may be passed to a URL via query parameters. This is commonly found on a search page where the search query is passed as a query parameter. These are the parts of the URL after a ?
, like /search?query=flask
. Flask makes it easy to access query parameters with the request.args
dictionary, for example:
@app.route('/search') def search(): query = request.args.get('query') # Meilisearch # See: https://github.com/meilisearch/meilisearch-python result = index.search(query) if query: return render_template('search.html', result=result) return 'No search query provided.'
In this case, when a user visits /search?query=flask
, we take the query and use it to retrieve the search result, which is then passed to the search.html
template for rendering.
Handling JSON Data
When building an API, we need the data delivered in JSON format. Flask provides a simple way to handle JSON data in requests with the jsonify
function. Here’s an example of handling JSON data:
from flask import jsonify @app.route('/api/data') def api_data(): return make_response(jsonify({"message": 'Success'}), 200)
Handling File Uploads
Flask also makes handling file uploads easy, using the request.files
object.
@app.route('/upload', methods=['GET', 'POST']) def upload_file(): if request.method == 'POST': file = request.files['file'] file.save(f'/uploads/{file.filename}') return redirect(url_for('index.html'))
In this example, when a user submits a file via the form, Flask saves the file to the specified directory and then redirects the user to the homepage.
Request Headers and Cookies
Sometimes you also need to get headers or cookies from the request in your app, such as for passing authentication or tracking user data. Flask provides easy access to headers through request.headers
and cookies through request.cookies
. Here’s a basic example of how we use it to authenticate for an API endpoint:
@app.route('/api/data') def check(): auth = request.headers.get('Authorization') nonce = request.cookies.get('nonce') # Simple authentication check if auth == 'Bearer X' and nonce == 'Y': return jsonify({"message": "Authenticated"}), 200 else: return jsonify({"message": "Unauthorized"}), 401
Wrapping up
Flask makes handling HTTP requests a breeze. Whether you’re working with basic GET
requests, handling form submissions with POST
, or dealing with more complex scenarios like JSON data and file uploads, it provides the APIs, functions, and tools you need to get the job done. We’ve only scratched the surface of Flask’s request-handling capabilities, but hopefully, this gives you a solid foundation to start building your own Flask apps.