Howdy: Modern WordPress Plugin Boilerplate
WordPress is a popular blogging platform built with PHP, and its extensibility is one of its greatest strengths. While you can create a plugin by dropping a single PHP file into your wp-content/plugins
directory, the broader development practices for WordPress plugins haven’t evolved much over the years-even as PHP itself has improved significantly.
PHP has changed significantly with new features and syntax. For example, it now includes better and proper OOP features and autoloading. However, WordPress still promotes the old procedural programming approach, and it’s not straightforward to include autoloading in your plugin.
This is why I created Howdy, a WordPress plugin boilerplate that aims to make using modern PHP concepts in WordPress plugin development easier.
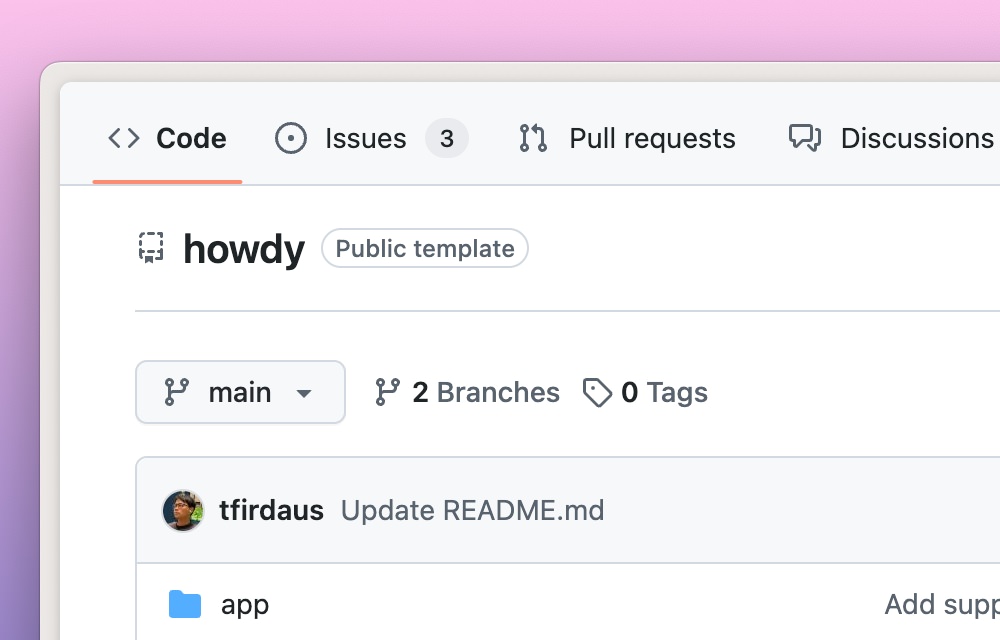
Scope
Howdy focuses on essential tools that improve productivity without overcomplicating the workflow. Rather than forcing every modern PHP practice into it, it prioritizes two foundational features:
- Namespacing
- Autoloading (with Composer)
Namespacing
Namespacing in PHP organizes classes, functions, and constants into logical groups, similar to how folders structure files, to prevent naming collisions. For example, two plugins defining a Security
class won’t conflict if each uses a unique namespace.
Traditionally, WordPress relies on prefixes for isolation. Suppose your plugin is named “Simple Security by Acme.” You’d typically prefix functions and classes with your organization name Acme_
or acme_
:
// Prefix in a function name. function acme_check_security() { // Add security check logic here } // Prefix in a class name. class Acme_Security { public function check() { // Add class-specific logic here } }
While namespaces can be used in WordPress plugins, adoption remains rare. This is because you can’t use namespaces to their full potential without autoloading.
Autoloading
Autoloading classes in WordPress has two key limitations.
First, you can’t autoload third-party libraries, like openai-php/client, without prefixing their namespaces. If two plugins load the same library, conflicting definitions will crash the site.
Additionally, without using Composer, all functions, constants, or static files must be manually loaded with require_once
, increasing boilerplate.
These are the two main issues that Howdy aims to tackle.
Once we have these two features properly set up, adopting other advanced PHP patterns, such as dependency injection or facades, becomes much easier.
So let’s install Howdy and see it in action.
Installation
We can install Howdy with the Composer create-project
command:
composer create-project syntatis/howdy -s dev
This command will create a new directory, howdy
, pull all the project files, and install the dependencies from Packagist.
If you want to create the project in a different folder, you can add the directory name at the end of the command, like below:
composer create-project syntatis/howdy -s dev acme-plugin
It will then ask you to input the plugin slug. The plugin slug is required and should be unique. If you plan to publish your plugin on WordPress.org, this slug will be used in the plugin URL, e.g., https://wordpress.org/plugins/{slug}/
. For this example, we’ll use acme-plugin
.
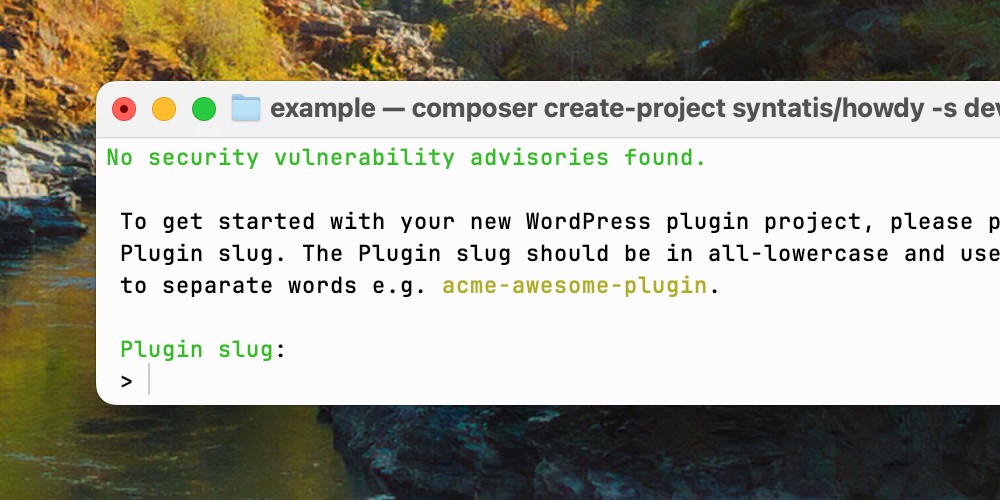
The plugin slug will also be used to determine the default plugin name, the namespace prefix, and more. As we can see below, it’s smart enough to transform the slug into the appropriate format. For this example, we’ll keep the default plugin name while changing the namespace to Acme
instead of AcmePlugin
.
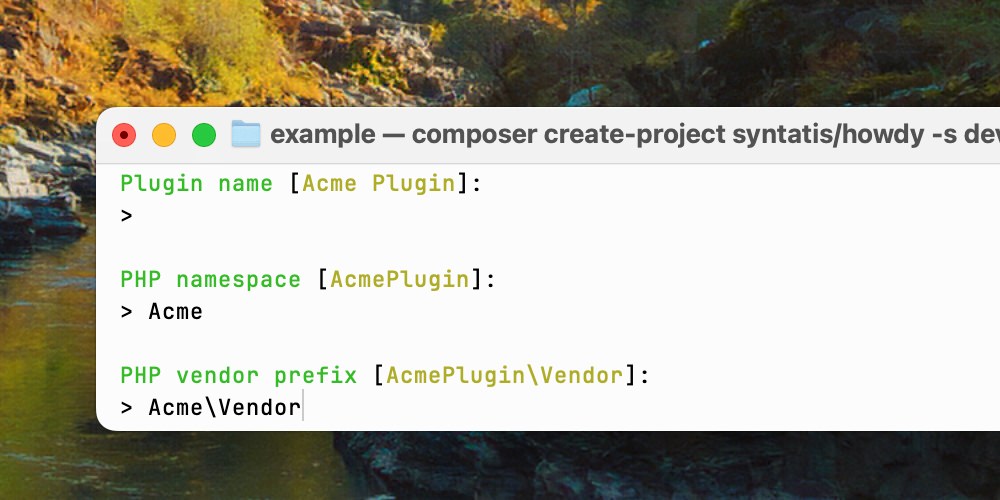
Once the inputs are completed, the necessary updates are made to the project files. For example, the file in app/Plugin.php
will include the namespace and the prefix for the dependencies’ namespace.
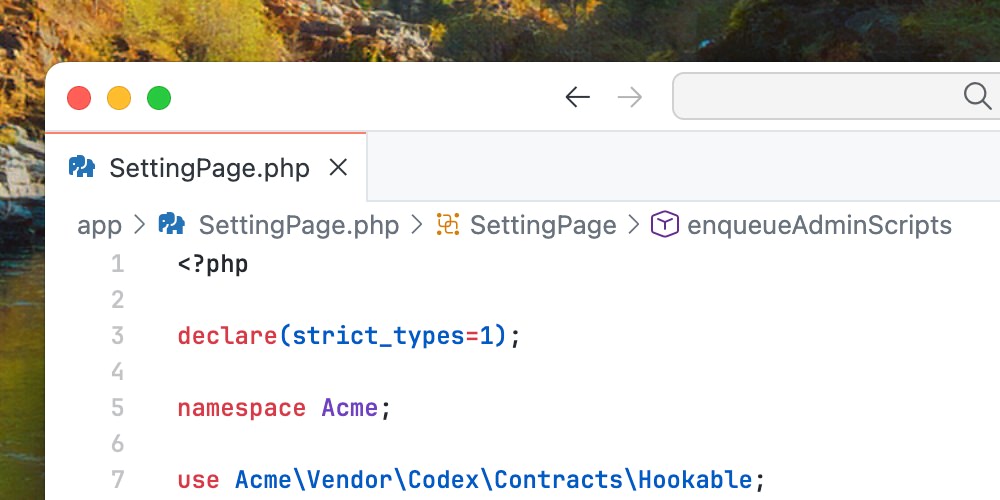
What’s Included?
Howdy comes pre-configured with these tools to streamline development:
- PHP-Scoper: It allows us to add prefixes for dependencies installed with Composer to prevent conflicts when using the same libraries.
- PHPCS: It includes PHPCS, but instead of using the WordPress Coding Standard, the library applies modern coding standards that are well-established in the PHP ecosystem, such as PSR-12, Doctrine, and Slevomat.
- Kubrick: A collection of React.js components to build applications like the settings page in the WordPress admin.
- @wordpress/scripts: To compile JavaScript and stylesheets. You can run the following command to start watching files and automatically compile changes:
npm run start
Directory Structure
Howdy uses a slightly unconventional structure for a WordPress plugin. However, if you’re familiar with frameworks like Laravel or Symfony, you’ll adapt quickly.
There are three main directories:
- app: This directory should house your plugin’s core classes and business logic. Classes in this directory are autoloaded automatically if they follow the PSR-4 standard.
- inc: This directory contains configuration files, utilities, and HTML templates.
- src: This directory contains the uncompiled source for JavaScript and stylesheet files.
Installing External Dependencies
Now that we have the plugin boilerplate set up, we can easily install additional packages with Composer. For example, if we want to build a plugin with OpenAI integration, we can include the openai-php/client
package using the following command:
composer require openai-php/client
Howdy will automatically add a prefix to the namespaces of all classes in this package after it’s installed.
You can also install packages specifically for development. For example, to install symfony/var-dumper, a popular PHP package for debugging, you can run:
composer require symfony/var-dumper --dev
This package provides a more user-friendly debugging experience compared to PHP’s native var_dump
function.
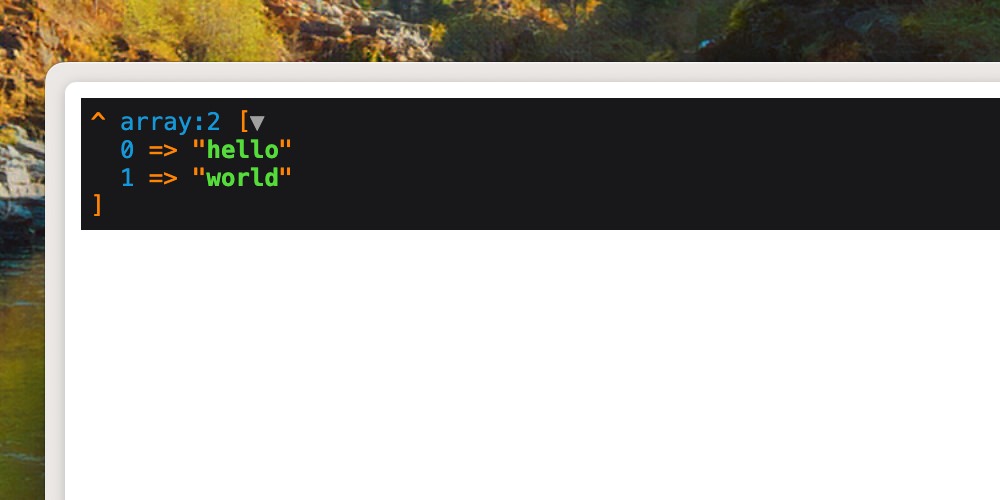
Preparing for Production
Lastly, Howdy provides several commands to prepare your plugin for release:
npm run build
: Builds all asset files, including JavaScript and stylesheets, in the src
directory. These files are optimized and minified for production.
composer run build
: Re-compiles the project and removes packages installed for development.
composer run plugin:zip
: Creates an installable ZIP file for the plugin. Unnecessary files like dotfiles, src
directories, and node_modules
are excluded from the final archive.
Wrapping Up
In this article, we’ve explored Howdy and its benefits for WordPress plugin development.
Howdy is designed to modernize plugin development without overwhelming you. It avoids bloating your workflow with every trendy tool (e.g., PHPUnit, PHPStan, or TypeScript are not included by default), but you can add them later as needed.
By solving dependency conflicts and enabling Composer, Howdy bridges WordPress development with the broader PHP ecosystem, unlocking countless possibilities for building maintainable and scalable plugins.