Understanding REST and How to Develop RESTful APIs
Web developers frequently discuss REST principles and RESTful data architecture, as they are essential components of modern development. However, the concepts can sometimes be confusing. REST is not a technology but rather a method for creating APIs based on specific organizational principles. These principles guide developers to create a more universal environment for processing API requests.
In this post, I will explain RESTful development practices from a high-level perspective, focusing on the what rather than the how. While I will touch on both aspects, this post is designed for web developers who are interested in REST APIs but find the concept difficult to grasp.
REST for Web Developers
The acronym REST stands for Representational State Transfer. This term might seem confusing, and the wiki entry may add to the confusion. However, the terminology can be simplified.
REST is essentially a set of guidelines and architectural styles used for data transmission. It is commonly applied to web applications but can also be used to pass data to software systems.
The acronym API stands for Application Programming Interface, which refers to methods of connecting with other libraries or applications. For instance, Windows has multiple APIs, and Twitter provides a web API. Although these APIs serve different purposes, they all follow the same underlying principles.
In summary, RESTful APIs are APIs that adhere to the REST architecture.
What Exactly is the REST Architecture?
While it can be challenging to define REST architecture precisely, some architectural constants include:
- Consistency across the entire API
- Stateless existence (i.e., no server-side sessions)
- Use of HTTP status codes where appropriate
- Use of URL endpoints with a logical hierarchy
- Versioning in the URL rather than in HTTP headers
There are no overly specific guidelines like the W3C’s HTML5 spec, which can sometimes lead to confusion and uncertainty around REST terminology.
The above list shouldn’t be considered hard-and-fast rules, although they are true for most modern RESTful APIs.
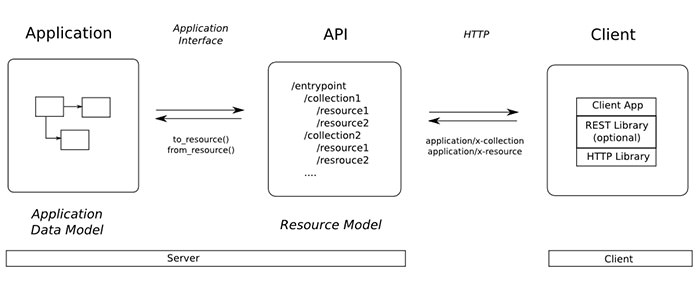
REST is a lightweight methodology, making it ideal for HTTP data transmission. This is why REST became so popular on the web and why it is widely regarded as the best choice for API development.
As Vinay Sahni aptly puts it, “an API is a developer’s UI.” Everything should be easy to use and provide an excellent user experience. RESTful APIs aim to achieve just that.
Key Takeaways for RESTful APIs
These tips are specific to APIs designed for web applications, meaning that HTTP is a requirement. Often, this also means that the API data is hosted on an external server. Let’s examine how RESTful APIs work from the perspective of the API user.
The API user, typically a web developer, can build a script that connects to an external API server. The necessary data is then transmitted over HTTP, allowing the developer to display data on their website without direct access to the external server (e.g., pulling Twitter data).
Generally, there are four main commands used to interact with RESTful APIs:
GET
for retrieving an objectPOST
for creating a new objectPUT
for modifying or replacing an objectDELETE
for removing an object
Each of these methods should be included with the API call to instruct the server on what action to take.
Most web APIs only allow GET
requests to retrieve data from an external server. While authentication is optional, it is highly recommended when allowing potentially destructive commands like PUT
or DELETE
.
However, not all RESTful APIs provide such extensive functionality. Consider Pokéapi, a free Pokémon API database. It is publicly accessible with decent rate limiting (limiting the number of API requests a user can make over time) but only allows the GET
method for accessing resources. This type of API may be referred to as a consumption-only API.
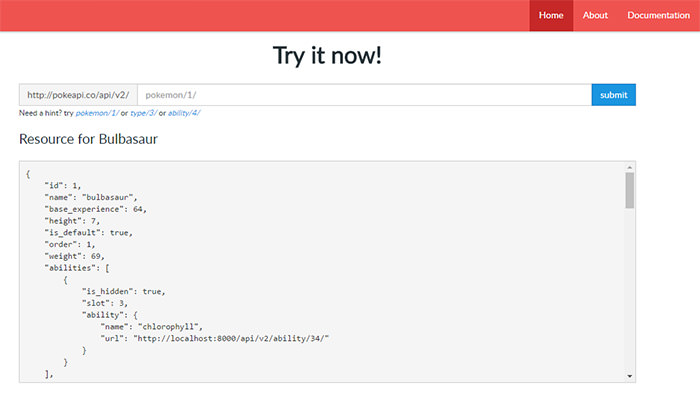
Maintaining consistency in return types is also important. JSON is a popular return type, and its specifications provide guidance on proper data structures.
RESTful APIs typically use nouns for API objects and verbs for actions performed on those objects. Authentication, rate limiting, and other security measures can be part of the API’s architecture, but a simple API can function without these additional complexities.
Accessing API Resources
Public APIs are typically accessible via direct website URLs. This makes URL structure critical and should be reserved exclusively for API requests.
Some URLs may include a prefix directory like /v2/
for an updated version 2 of a previous API. This approach is common among developers who want to offer a new API structure without deprecating their existing 1.x API.
I found this post particularly insightful regarding basic URL structures and examples from other services.
It’s important to note that the endpoint’s return data will vary significantly depending on the HTTP method. For example, GET
retrieves content, while POST
creates new content. The request might point to the same endpoint, but the result could be very different.
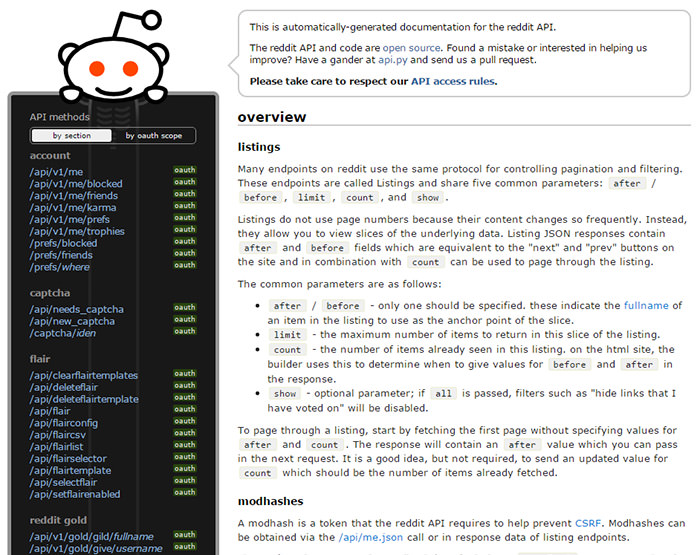
Reviewing examples online can help clarify concepts. We’ve already looked at the Pokéapi, but here are some other real-world API examples to explore:
Building Your Own API
Developing your own API is a significant undertaking, but it’s not as complicated as it might seem. It requires an understanding of API design patterns and best practices to create something of real value.
Each API must connect to your server to return data. In addition to writing code to achieve this, you also need to format the return data appropriately. Other potential requirements include authentication and rate limiting, so building an API is not a task to be taken lightly.
Let’s examine some fundamental principles of API architecture.
Build Endpoints
One of the essential aspects of API development is building endpoints. When creating resources, it’s important to use nouns, not verbs. This means API data should represent a person, place, or thingâÂÂmost often a thing with specific attributes (e.g., a tweet and all its metadata).
Learning to name nouns can be challenging, but it’s a crucial aspect of API development. Simplification is key whenever possible.
There’s an ongoing debate over singular vs. plural nouns. If you were creating a Twitter API, you might organize the object group first (e.g., tweet) and the object item second (e.g., tweet ID).
$ /tweet/15032934882934 $ /tweets/15032934882934
In this case, the singular form often looks better, especially when returning only one resource. However, there is no universally correct answer, so choose what works best for your project.
Set Return Type
Another important consideration is the return type data. Most web users expect JSON content, making it the best option. XML is another choice if you want to offer both. However, JSON is the preferred API return type among web developers.
There’s much more to API development, so I recommend exploring APIs to see how other developers build them. This experience will help you become familiar with typical requirements and best practices.
If you’re just starting out, consider these development tutorials:
Further Resources
The best way to learn web app development is through practice. While theory is valuable for understanding the underlying concepts, hands-on experience is essential for practical knowledge.
A good starting point for API development is connecting to existing APIs. Once you’ve mastered the basics of client-side connections, you can move on to server-side API development by building your own API from scratch.
If you’re ready to dive in, consider the following resources to guide you on your journey.
Books
- REST API Design Rulebook
- RESTful Web APIs
- RESTful Web Services Cookbook
- Undisturbed REST: A Guide to Designing the Perfect API