Easily Validate Form Inputs Across All Browsers with Validatr
Working with web forms can be both exciting and challenging. There’s plenty of room for customization in design, labels, and even authentication. With the numerous new features available in HTML5, Jay Morrow created Validatr, a tool that leverages the new HTML5 input attributes to enable easy and automatic form validation.
Validatr is a free, cross-browser jQuery plugin. It automatically highlights the form border in red if the user inputs an invalid value. Validatr supports various input validations, including email, number, URL, range, color inputs, and date.
Custom error messages are also available using HTML5 data attributes. You can style the error message element with your own CSS, and the plugin comes with several pre-designed CSS styles.
Getting Started
To use Validatr, include the latest jQuery library and the Validatr script in your project.
<script src="http://code.jquery.com/jquery-latest.min.js" type="text/javascript"></script> <script src="js/validatr.js" type="text/javascript"></script>
Then, call the plugin with the following snippet:
<script> jQuery(function ($) { $('form').validatr(); }); </script>
The plugin will automatically apply changes to the form
element.
HTML Markup
As mentioned, Validatr utilizes the input
element within the form
tag. The input
element will handle various form types and settings using HTML5 data-attributes
.
For example, to create a number input form, simply add type="number"
to the input
element and include additional parameters like min and max values. If users are required to fill out the form, include the required
attribute.
The following is a basic implementation example:
<form action="./"> <label for="number">Number</label> <div> <input type="number" id='number' step=2 min=1 max=11 name="number" required> <input type="submit" value="Submit"> </div> </form>
The step
attribute controls the value increments when the user clicks the increase or decrease arrow in the form, starting from the defined min
number or 0. If the value is set to “0”, it uses the default increment of one point (the value must be a positive number).
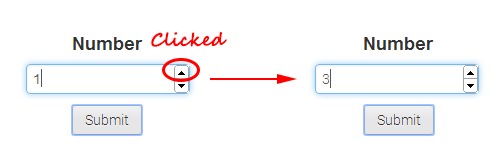
Here’s what happens when a user inputs an invalid value or a number outside the specified range. In our example, with a step
of 2 starting from 1, the only valid numbers are 3, 5, 7, etc., up to the max value of 11.
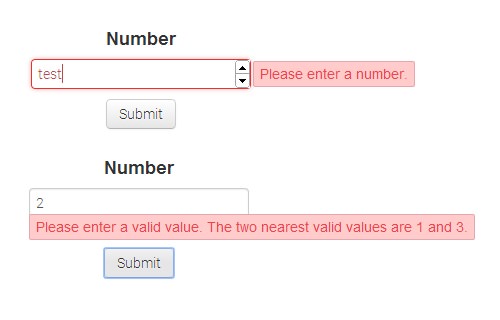
For a complete list of supported input types and attribute documentation, please visit the Validatr page.